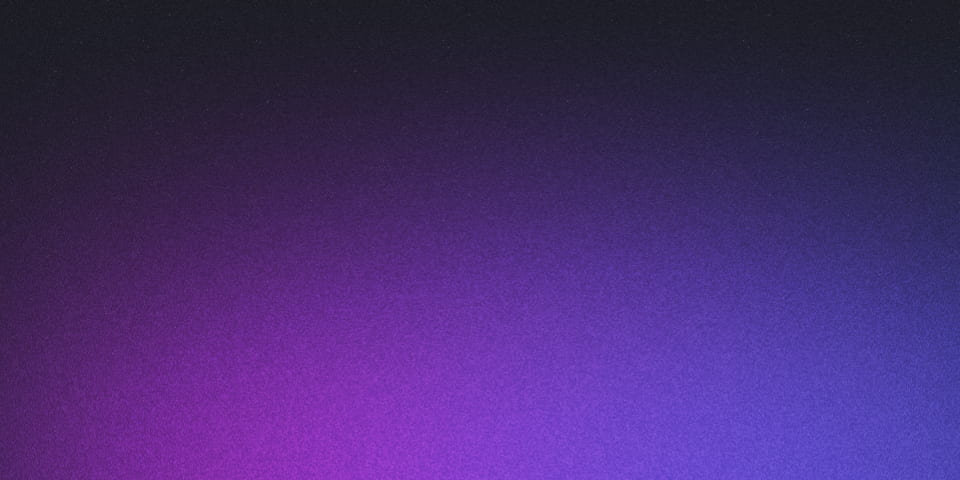
Leaner React - Part 1 - Smaller Components
For a long time, I’ve been wanting to write about an opinionated approach of writing leaner React/JSX. React provides a great developer experience and making it trivial to write web applications. However, it is sometimes too easy, and when you don’t follow somes rules to keep your code clean, it becomes a mess very fast. Let’s get into it!
Disclaimer: these are not hard rules, but rather recommendations that usually lead to cleaner code. There are exceptions that justify not following them.
TLDR:
- Avoid big components, prefer small components
- Avoid long, confusing names, prefer short/descriptive names
- Avoid smart components, prefer dumb components
- Avoid Higher-Order Components (HOCs), prefer hooks, props or children
- Avoid named props for content, prefer children
- Avoid repetition, prefer map()
- Avoid inlining/declaring functions inside JSX, prefer named functions
- Avoid unnecessary wrapper elements, prefer unwrapped elements or fragments
- Avoid start/end tags, prefer self-closing components (where possible)
Anatomy of a React component
A file with a React component is typically composed of following parts:
- imports: outside dependencies
- static definitions: constants & types
- component definition: the actual component
- component variables, hooks & functions: state, refs, effects etc.
- component markup: html-like template
- (Optional) helper functions: functions that don’t need to be recreated on every render
This translates to:
Component Anatomy
// 1. imports
import { useState, useEffect } from "react";
// 2. static definitions
const LIMIT = 10;
type CounterProps = {
initialState: number;
};
// 3. component definition
export function Counter({ initialState }: CounterProps) {
// 4. component variables, hoooks & functions
const [state, setState] = useState(initialState);
useEffect(() => {
if (isExceedingLimit(state)) {
setState(0);
}
}, [state]);
const increment = () => {
setState(state + 1);
};
// 5. component markup
return (
<div>
<button onClick={increment}>+1</button>
{state}
</div>
);
}
// 6. helper functions
function isExceedingLimit(state: number) {
return state > LIMIT;
}
This is a pretty common structure and already quite lean. However, there are recommendations for each part in this series, but we’ll start with the most important one: keep the whole file small. Small files can be understood in a few seconds, while large files take exponentially more time to understand.
Avoid big components, prefer small components
The often touted reason to extract code into its own component is to make it reusable. While this is a good reason to extract them, it is not the most important one in my opinion. Extracting code into own, well-named components is much more important. It’s the good ol’ divide and conquer principle. Many small components are easier to conquer (understand) than one big component. Let’s look at these two components:
Big component
export function Index() {
return (
<body>
<header>
<nav>
<ul>
<li>
<a>Home</a>
</li>
<li>
<a>About</a>
</li>
<li>
<a>Contact</a>
</li>
</ul>
</nav>
</header>
<main>
<article>
<h1>Hello World!</h1>
<p>This is just an example article on a website.</p>
</article>
</main>
<footer>
<p>© 2021</p>
<a>Imprint</a>
</footer>
</body>
);
}
Small Component
export function Index() {
return (
<body>
<Header />
<Main />
<Footer />
</body>
);
}
I think everybody would agree that the second is easier to understand. You might say “but that’s cheating! You’re just moving the code somewhere else!” That’s exactly the point. Most of the times you don’t need to see everything at once. Most often you only care about a very specific part at a time. And only seeing that part, without noise from other unrelated parts around it, reduces the cognitive load immensely.
Avoid long, confusing names, prefer short/descriptive names
Naming things are hard. But it’s worth the effort. A good name can make the difference between understanding a piece of code in a few seconds or minutes. A good name is descriptive and tells you what the component does. If you can’t come up with a good name, it’s a good indicator that the component is doing too much.
Avoid smart components, prefer dumb components
Components that contain a lot of declarations, business logic, rendering logic, and markup can be labeled as “smart” components. They usually are harder to understand and maintain. Instead, prefer “dumb” components that follow the single responsibility principle: do one thing and do it well.
Note: Single-purpose & reusability often collide, but don’t have to. A component can be single-purpose and still be reusable (e.g. a styled button). But as soon as a component has to fit different use-cases (=become smart), it’s a bad sign.
Avoid Higher-Order Components (HOCs), prefer hooks, props or children
HOCs are often confusing, increase the code complexity and are highly inefficient. Whatever problem you’re trying to solve, there is most often is a better alternative to HOCs.
Avoid named props for content, prefer children
When you have a small component, e.g. a button, and you want to make it reusable, you might be tempted pass its content via a named prop (e.g. label
, title
, name
etc.) that accepts string
or ReactNode
values . While this seems like a fitting solution, in the long run it makes components more complex.
Named Props
function Index() {
return (
<div>
<Button label="Hello World!" />
</div>
);
}
Instead, pass the content as children (just like normal html):
Children Props
function Index() {
return (
<div>
<Button>Hello World!</Button>
</div>
);
}
Avoid repetition, prefer map()
When you have a list of items that you want to render, you might write the same code over and over again:
Repeated Content
function Index() {
return (
<header>
<nav>
<ul>
<li>
<a>Home</a>
</li>
<li>
<a>About</a>
</li>
<li>
<a>Contact</a>
</li>
</ul>
</nav>
</header>
);
}
Prefer using map()
to render repeating elements:
Mapped Content
const items = ["Home", "About", "Contact"];
function Index() {
return (
<header>
<nav>
<ul>
{items.map((item) => (
<li key={item}>{item}</li>
))}
</ul>
</nav>
</header>
);
}
Avoid inlining/declaring functions inside JSX, prefer named functions
While inline functions are convenient and okayish if they fit in one line. Beyond that, it makes the markup harder to read and understand - especially if you have nested blocks. Prefer named functions that are declared before the return statement. If you seek better performance, declare them outside of the component.
Avoid unnecessary wrapper elements, prefer unwrapped elements or fragments
Have you ever seen a component that looks like this?
Wrapped Element
function Index() {
return (
<div className="flex items-center justify-center">
<div className="flex items-center justify-center">
<div className="flex items-center justify-center">Hello World!</div>
</div>
</div>
);
}
Prefer less elements (and if possible, prefer fragments <></>
):
Unwrapped Element
function Index() {
return <div className="flex items-center justify-center">Hello World!</div>;
}
This is also valid for libraries that wrap other libraries (which were originally written for vanilla javascript), like react-map-gl
(mapbox/maplibre wrapper), recharts
(d3 wrapper), etc. Avoid them if possible and prefer the original library.
Avoid start/end tags, prefer self-closing components (where possible)
When a component doesn’t have children, prefer self-closing components. This is also available as linting rule: react/self-closing-comp
Start and end tag
function Index() {
return (
<>
<Header></Header>
<Main></Main>
<Footer></Footer>
</>
);
}
Self-closing tag
function Index() {
return (
<>
<Header />
<Main />
<Footer />
</>
);
}
Conclusion
That’s it for part 1! Applying these recommendations will significantly reduce the complexity of your files / components. In the next part, we’ll dive into state management and keep React components as stateless as possible.